GET /rest/agile/1.0/board Returns all boards. This only includes boards that the user has permission to view. RequestQuery parametersmaxResults integer Format: int32 name string projectKeyOrId string type StringList startAt integer Format: int64 Example
curl --request GET \
--url 'http://{baseurl}/rest/agile/1.0/board' \
--user 'email@example.com:<api_token>' \
--header 'Accept: application/json' |
# This code sample uses the 'requests' library:
# http://docs.python-requests.org
import requests
from requests.auth import HTTPBasicAuth
import json
url = "http://{baseurl}/rest/agile/1.0/board"
auth = HTTPBasicAuth("email@example.com", "<api_token>")
headers = {
"Accept": "application/json"
}
response = requests.request(
"GET",
url,
headers=headers,
auth=auth
)
print(json.dumps(json.loads(response.text), sort_keys=True, indent=4, separators=(",", ": "))) |
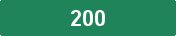
Returns the requested boards, at the specified page of the results. 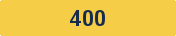
Returned if the request is invalid. A schema has not been defined for this response code. 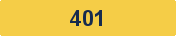
Returned if the user is not logged in. A schema has not been defined for this response code.
|
POST /rest/agile/1.0/board Creates a new board. Board name, type and filter Id is required. - name - Must be less than 255 characters.
- type - Valid values: scrum, kanban
- filterId - Id of a filter that the user has permissions to view. Note, if the user does not have the 'Create shared objects' permission and tries to create a shared board, a private board will be created instead (remember that board sharing depends on the filter sharing). Note:
- If you want to create a new project with an associated board, use the JIRA platform REST API. For more information, see the Create project method. The projectTypeKey for software boards must be 'software' and the projectTemplateKey must be either com.pyxis.greenhopper.jira:gh-kanban-template or com.pyxis.greenhopper.jira:gh-scrum-template.
- You can create a filter using the JIRA REST API. For more information, see the Create filter method.
- If you do not ORDER BY the Rank field for the filter of your board, you will not be able to reorder issues on the board.
RequestBody parametersfilterId integer Format: int64 name string type string curl --request POST \
--url 'http://{baseurl}/rest/agile/1.0/board' \
--user 'email@example.com:<api_token>' \
--header 'Accept: application/json' \
--header 'Content-Type: application/json' |
# This code sample uses the 'requests' library:
# http://docs.python-requests.org
import requests
from requests.auth import HTTPBasicAuth
import json
url = "http://{baseurl}/rest/agile/1.0/board"
auth = HTTPBasicAuth("email@example.com", "<api_token>")
headers = {
"Accept": "application/json",
"Content-Type": "application/json"
}
response = requests.request(
"POST",
url,
headers=headers,
auth=auth
)
print(json.dumps(json.loads(response.text), sort_keys=True, indent=4, separators=(",", ": "))) |
|
GET /rest/agile/1.0/board/{boardId} Returns a single board, for a given board Id. RequestPath parametersboardId Required integer Format: int64 Example
curl --request GET \
--url 'http://{baseurl}/rest/agile/1.0/board/{boardId}' \
--user 'email@example.com:<api_token>' \
--header 'Accept: application/json' |
# This code sample uses the 'requests' library:
# http://docs.python-requests.org
import requests
from requests.auth import HTTPBasicAuth
import json
url = "http://{baseurl}/rest/agile/1.0/board/{boardId}"
auth = HTTPBasicAuth("email@example.com", "<api_token>")
headers = {
"Accept": "application/json"
}
response = requests.request(
"GET",
url,
headers=headers,
auth=auth
)
print(json.dumps(json.loads(response.text), sort_keys=True, indent=4, separators=(",", ": "))) |
|
GET /rest/agile/1.0/board/{boardId}/backlog Returns all issues from a board's backlog, for a given board Id. RequestPath parametersboardId Required integer Format: int64 Query parametersexpand string jql string maxResults integer Format: int32 validateQuery boolean fields Array<StringList> startAt integer Format: int64 Examplecurl --request GET \
--url 'http://{baseurl}/rest/agile/1.0/board/{boardId}/backlog' \
--user 'email@example.com:<api_token>' \
--header 'Accept: application/json' |
|
GET /rest/agile/1.0/board/{boardId}/epic Returns all epics from the board, for the given board Id. This only includes epics that the user has permission to view. Note, if the user does not have permission to view the board, no epics will be returned at all. RequestPath parametersboardId Required integer Format: int64 Query parametersmaxResults integer Format: int32 done string startAt integer Format: int64 Examplecurl --request GET \
--url 'http://{baseurl}/rest/agile/1.0/board/{boardId}/epic' \
--user 'email@example.com:<api_token>' \
--header 'Accept: application/json' |
# This code sample uses the 'requests' library:
# http://docs.python-requests.org
import requests
from requests.auth import HTTPBasicAuth
import json
url = "http://{baseurl}/rest/agile/1.0/board/{boardId}/epic"
auth = HTTPBasicAuth("email@example.com", "<api_token>")
headers = {
"Accept": "application/json"
}
response = requests.request(
"GET",
url,
headers=headers,
auth=auth
)
print(json.dumps(json.loads(response.text), sort_keys=True, indent=4, separators=(",", ": "))) |
|
GET /rest/agile/1.0/board/{boardId}/epic/none/issue Returns all issues that do not belong to any epic on a board, for a given board Id. RequestPath parametersboardId Required integer Format: int64 Query parametersexpand string jql string maxResults integer Format: int32 validateQuery boolean fields Array<StringList> startAt integer Format: int64 Example# This code sample uses the 'requests' library:
# http://docs.python-requests.org
import requests
from requests.auth import HTTPBasicAuth
import json
url = "http://{baseurl}/rest/agile/1.0/board/{boardId}/epic/none/issue"
auth = HTTPBasicAuth("email@example.com", "<api_token>")
headers = {
"Accept": "application/json"
}
response = requests.request(
"GET",
url,
headers=headers,
auth=auth
)
print(json.dumps(json.loads(response.text), sort_keys=True, indent=4, separators=(",", ": "))) |
|
|